
#Expressions meaning code#
Place the code where an exception might occur inside a try block. Use the try-catch statement to handle exceptions that might occur during execution of a code block. You can use the try statement in any of the following forms: try-catch - to handle exceptions that might occur during execution of the code inside a try block, try-finally - to specify the code that is executed when control leaves the try block, and try-catch-finally - as a combination of the preceding two forms. Throw new InvalidCastException("Conversion to a DateTime is not supported.") The following example uses a throw expression to throw an InvalidCastException to indicate that a conversion to a DateTime value is not supported: DateTime ToDateTime(IFormatProvider provider) => Throw new ArgumentNullException(paramName: nameof(value), message: "Name cannot be null") Īn expression-bodied lambda or method. The following example uses a throw expression to throw an ArgumentNullException when the string to assign to a property is null: public string Name : throw new ArgumentException("Please supply at least one argument.") The following example uses a throw expression to throw an ArgumentException when the passed array args is empty: string first = args.Length >= 1 This might be convenient in a number of cases, which include: For more information, see the How exceptions are handled section of the C# language specification. If no catch block is found, the CLR terminates the executing thread. If the currently executed method doesn't contain such a catch block, the CLR looks at the method that called the current method, and so on up the call stack. When an exception is thrown, the common language runtime (CLR) looks for the catch block that can handle this exception. Opposite to that, throw e updates the StackTrace property of e.
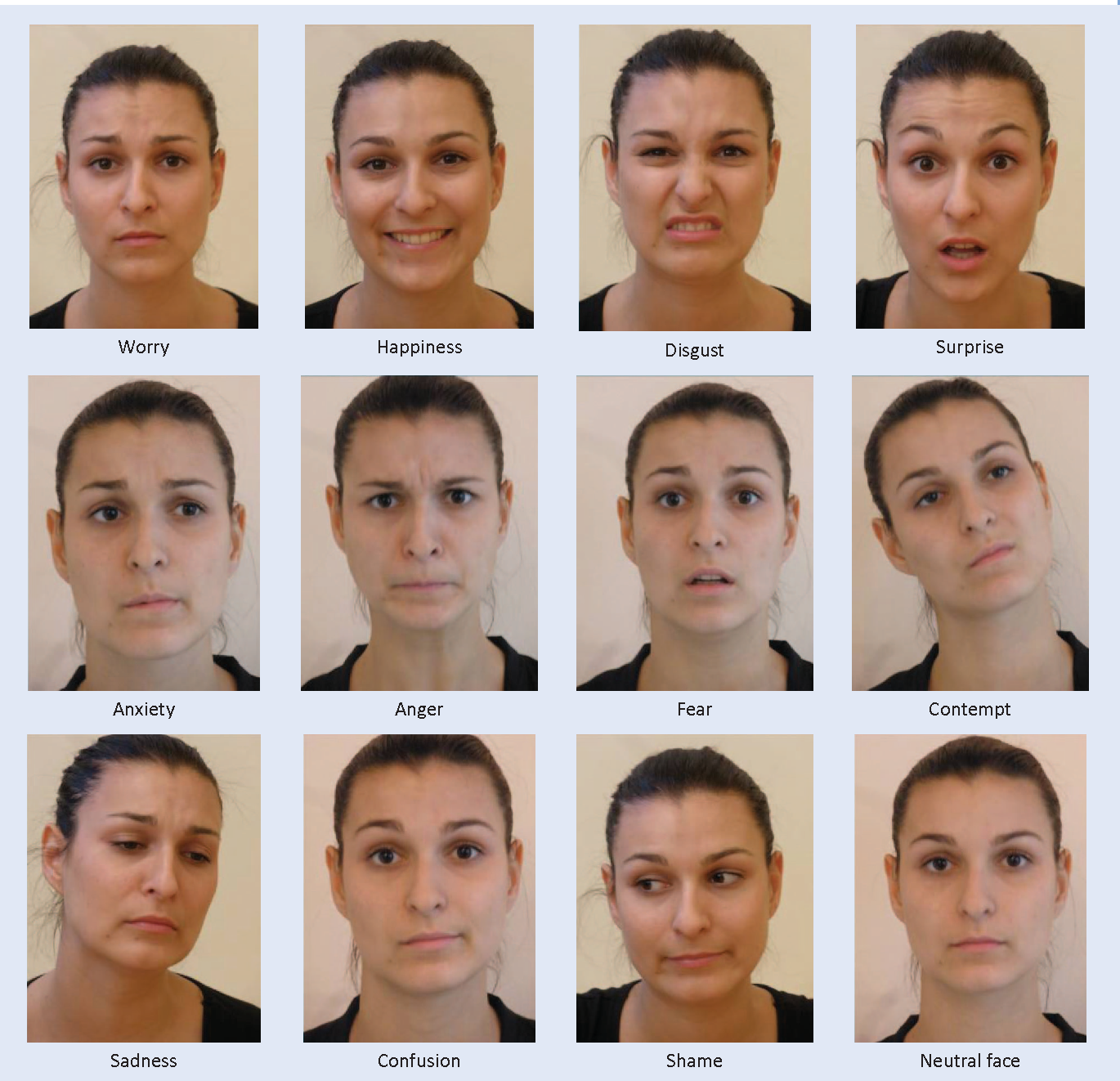
Throw preserves the original stack trace of the exception, which is stored in the Exception.StackTrace property. Inside a catch block, you can use a throw statement to re-throw the exception that is handled by the catch block: try For more information, see Creating and throwing exceptions. You can also define your own exception classes that derive from System.Exception.

You can use the built-in exception classes, for example, ArgumentOutOfRangeException or InvalidOperationException.NET also provides the helper methods to throw exceptions in certain conditions: ArgumentNullException.ThrowIfNull and ArgumentException.ThrowIfNullOrEmpty. In a throw e statement, the result of expression e must be implicitly convertible to System.Exception. Throw new ArgumentOutOfRangeException(nameof(shapeAmount), "Amount of shapes must be positive.") The throw statement throws an exception: if (shapeAmount <= 0) Use the try statement to catch and handle exceptions that might occur during execution of a code block. Use the throw statement to throw an exception. You use the throw and try statements to work with exceptions.
